server.js
const http = require('http');
const hostname = '0.0.0.0';
const port = 1337;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.end('Hello World!');
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
server.php
<?php
require __DIR__ . '/vendor/autoload.php';
error_reporting(-1);
use Amp\Http\Server\RequestHandler\CallableRequestHandler;
use Amp\Http\Server\Server;
use Amp\Http\Server\Request;
use Amp\Http\Server\Response;
use Amp\Http\Status;
use Amp\Socket;
use Psr\Log\NullLogger;
// Run this script, then visit http://localhost:1337/ in your browser.
Amp\Loop::run(function () {
$sockets = [
Socket\listen("0.0.0.0:1338"),
];
$server = new Server($sockets, new CallableRequestHandler(function (Request $request) {
return new Response(Status::OK, [
], "Hello World!");
}), new NullLogger());
yield $server->start();
});
swoole.php
<?php
$http = new swoole_http_server("127.0.0.1", 9501);
$http->on("start", function ($server) {
echo "Swoole http server is started at http://127.0.0.1:9501\n";
});
$http->on("request", function ($request, $response) {
$response->end("Hello World");
});
$http->start();
server.go
package main
import (
"fmt"
"log"
"net/http"
)
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintf(w, "Hello World")
}
func main() {
http.HandleFunc("/", handler)
log.Fatal(http.ListenAndServe(":8080", nil))
}
测试命令为:ab -c1000 -n30000 http://127.0.0.1:1337/
最后结果对比如下:
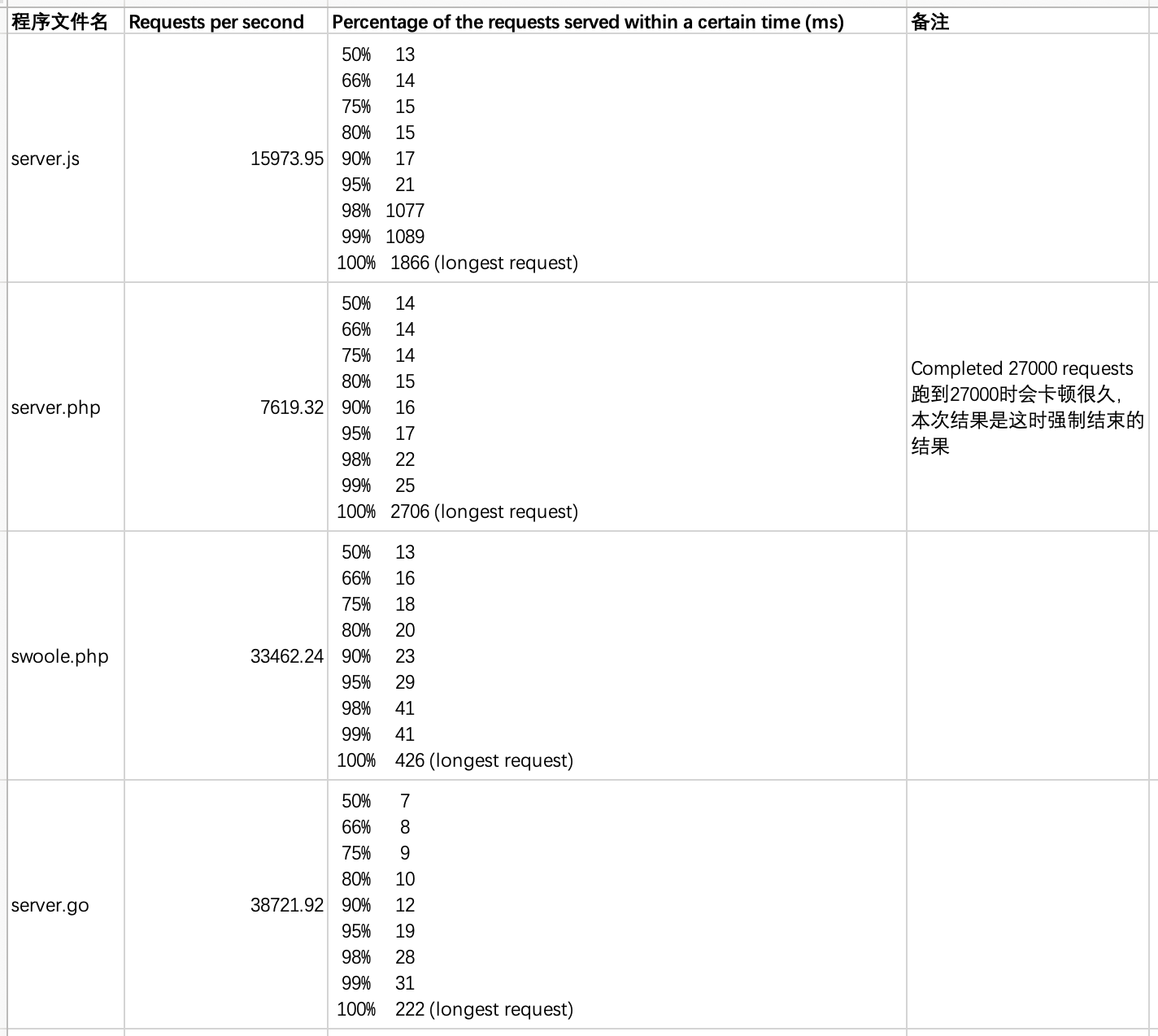